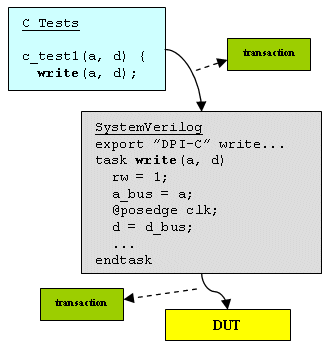
Introduction
The e language has a number of predefined data types including the integer and Boolean scalar types common to most programming languages. Below is list of data types that we will be seeing in detail in next few pages. As such e language data types are far better then what we find in SystemC and TestBuilder (man I hate type conversions).
- Scalar Types
- Scalar Subtypes
- Enumerated Scalar Types
- Struct Types
- Struct Subtypes
- List Types
- The String Type
Scalar Data Types
Scalar data types are one of the below
- Numeric
- Boolean
- Enumerated
Numeric and Boolean Scalar Types | ||
Below Table shows the e Numeric and Boolean scalar types | ||
 | ||
Type Name | Function | Â |
int | Represents numeric data, both negative and non-negative integers. Default Size = 32 bits | Â |
uint | Represents unsigned numeric data, non-negative integers only. Default Size = 32 bits | Â |
bit | An unsigned integer in the range 0-1. Size = 1 bit | Â |
byte | An unsigned integer in the range 0-255. Size = 8 bits | Â |
time | An integer in the range 0-263-1. Default Size = 64 bits | Â |
bool | Represents truth (logical) values, TRUE (1) and FALSE (0). Default Size = 1 bit | Â |
Example |
1 <'
2 struct data_types1 {
3 // Signed Integer Date Type
4 memory_address : int;
5 // Unsigned Integer Data Type
6 memory_data : uint;
7 // Bit Data Type
8 chip_enable : bit;
9 // Byte Data Type
10 read_data : byte;
11 // Time Data Type
12 current_time : time;
13 // Boolean Data Type
14 };
15 '>
Scalar Sub-Types
You can create a scalar subtype by using a scalar modifier to specify the range or bit width of a scalar type. You can also specify a name for the scalar subtype if you plan to use it repeatedly in your program. Unbounded integers are a predefined scalar subtype.
- int [0..100] (bits: 7)
- int [0..100] (bits: 7)
- int (bytes: 1)
- int (bits: 8)
Example
1 <'
2
3 type valid_range_a : int [0..100] (bits:8);
4 type valid_range_d : int [0..150] (bytes:1);
5
6 struct data_types2 {
7 // Signed Integer Date Type
8 memory_address : int (bits:8);
9 // Unsigned Integer Data Type
10 memory_data : uint (bits:8);
11 // Valid range with bits
12 read_address : valid_range_a;
13 // Valid range with byte
14 read_data : valid_range_d;
15 };
16 '>
Enumerated Scalar Types
As in any programming language, we can have enumerated data types in e language also, Below is the list of things for a e language enumerated scalar types. |
- You can define the valid values for a variable or field as a list of symbolic constants.
- These symbolic constants have associated unsigned integer values.
- By default, the first name in the list is assigned the value zero.
- Subsequent names are assigned values based upon the maximum value of the previously defined enumerated items + 1.
- You can also assign explicit unsigned integer values to the symbolic constants.
- We can define named enumerated type as an empty type, This can be later extended.
Example
1 <'
2 type kind_default_values : [good, bad];
3 type kind_assigned_values : [good = 2, bad = 3];
4 type kind_only_few : [good = 3, bad];
5 // Empty
6 type packet_protocol: [];
7 // Extend
8 extend packet_protocol : [Ethernet, IEEE, foreign];
9 //Sized
10 type packet_kind: [good, bad] (bits: 2);
11
12 struct data_types3 {
13 packet_type : packet_protocol;
14 packet_valid : packet_kind;
15 };
16 // Just to check our code
17 extend sys {
18 data : data_types3;
19 run() is also {
20 gen data;
21 print data;
22 gen data;
23 print data;
24 gen data;
25 print data;
26 };
27 };
28 '>
data = data_types3-@0: data_types3
---------------------------------------------- @data_types3
0 packet_type: foreign
1 packet_valid: good
data = data_types3-@1: data_types3
---------------------------------------------- @data_types3
0 packet_type: foreign
1 packet_valid: bad
data = data_types3-@2: data_types3
---------------------------------------------- @data_types3
0 packet_type: Ethernet
1 packet_valid: good
Bạn Có Đam Mê Với Vi Mạch hay Nhúng - Bạn Muốn Trau Dồi Thêm Kĩ Năng
Â